How to programmatically navigate using React Router
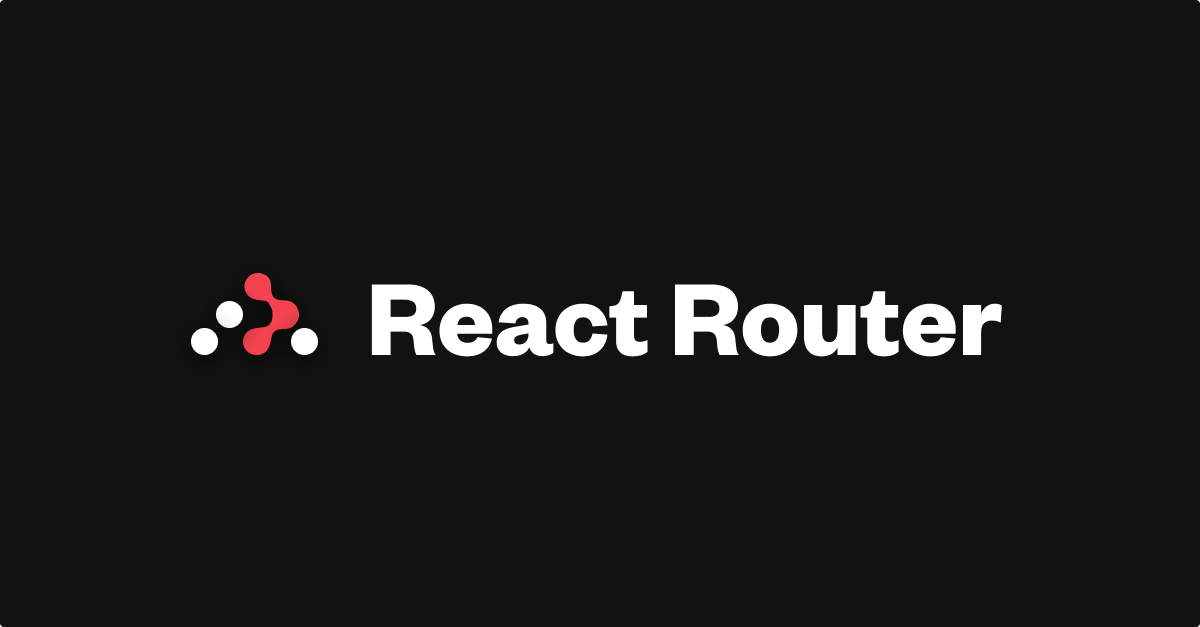
React Router is a key library for adding navigation in React applications. This guide covers the essentials of programmatically navigating using React Router.
Setup
First, install React Router:
npm install react-router-dom
Wrap your application in <BrowserRouter>
:
import { BrowserRouter as Router } from 'react-router-dom';
function App() {
return (
<Router>
{/* Routes go here */}
</Router>
);
}
Defining Routes
Use <Route>
to map paths to components:
import { Route } from 'react-router-dom';
<Route path="/about" component={About} />
Programmatic Navigation
For programmatically navigating, use the useNavigate
hook:
import { useNavigate } from 'react-router-dom';
function MyComponent() {
let navigate = useNavigate();
function handleClick() {
navigate('/home'); // Navigate to home
}
return <button onClick={handleClick}>Go to Home</button>;
}
Navigating with Parameters
Pass parameters and state like this:
navigate('/user/123', { state: { from: 'MyComponent' } });
Redirects
Use <Navigate>
for redirection:
import { Navigate } from 'react-router-dom';
<Route path="/old-path" element={<Navigate to="/new-path" />} />
Conclusion
React Router offers a simple way to implement navigation in React apps. For more details, refer to the React Router documentation.